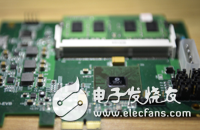
The first chapter is the basis of programming. This article is a 1.8.1 character constant.
>>> Â 1. Â Reference to a character constant
Character constants are surrounded by a pair of single quotes "'', for example, 'O', 'the compiler knows that this symbol refers to the ASCII value of the letter O, which is 79. You can also use ' ' to indicate a space, or use '9' to indicate the number 9. The constant '9' refers to a character and should not be confused with an integer value of 9. Unless the programmer can remember the ASCII table, anyone who sees 79 will not associate the letter O, and the character constant 'O' can directly convey its meaning.
In C, characters can be evaluated like integers without special conversion. Based on this, you can add an integer to a character. For example, the character c is added to the integer n, that is, c+n represents the nth character after c. It is also possible to subtract an integer from a character, for example, the expression cn represents the nth character before c. You can also subtract another character from one character. For example, c1 and c2 are both characters, then c1-c2 represents the distance between two characters.
Furthermore, you can compare two characters, if in the ASCII table, c1 is in front of c2, then c1
If( ch >= '0' && ch <= '9' ) { ... }
This separates the numeric characters from the other characters in the ASCII table. Although the standard C interface ctype.h provides the corresponding functions, if you implement them from start to finish, it will help you further understand their operation. If ch is an uppercase letter, return its corresponding lowercase letter, otherwise return ch itself, as shown in Listing 1.35.
Program Listing 1.35 tolower() function sample program
1 char tolower(char ch)
2 {
3 if( ch >= 'A' && ch <= 'Z' ){ // Â Identify uppercase letters
4 return (ch + ('a' - 'A'));
5 }else{
6 return (ch);
7 }
8 }
>>> Â 2. Character input and output
Although the convert %c allows the scanf() and printf() functions to read and write to a single character. such as:
Char ch;
Scanf("%c", &ch);
Printf("%c", ch);
But before reading the characters, the scanf() function does not skip the space character, which reads the space as a character into the variable ch. In order to solve this problem, you must add a space in front of %c:
Scanf(" %c", &ch);
Although the scanf() function does not skip the space character, it is easy to detect if the character being read is a newline character ''. such as:
While(ch != ''){
Scanf("%c", &ch);
}
Of course, you can also call getchar() and putchar() to read and write a single character. These are the macros defined in stdio.h, which are used to read data from the keyboard and print characters to the screen. Although macros and functions have some technical differences, their usage is the same. such as:
Int getchar(void); // Â Enter one character
Int putchar(int ch); // Â Output one character
The getchar() function takes no arguments and returns a character from the input queue. For example, the following statement reads a character input and assigns the value of the character to the variable ch:
Ch = getchar();
This statement is equivalent to the following statement:
Scanf("%c", &ch);
The putchar() function prints its arguments. For example, the following statement prints the value previously assigned to ch as a character:
Putchar(ch);
This statement has the same effect as the following statement:
Printf("%c", ch);
Since these functions only process characters, they are faster than the scanf() and printf() functions, which are usually defined in stdio.h, which are actually preprocessor macros, not real functions. Although these macros seem simple, sometimes there are problems, but there is no reason. such as:
Char ch1, ch2;
Ch1 = getchar();
Ch2 = getchar();
Printf("%d %d", ch1, ch2);
At this time, if the character 'a' is input, the print result is "97, 10". Since a result is printed after inputting a character from the keyboard, the program has not been entered yet. Since the keyboard input is stored at a time, the data will be stored in a temporary storage area called a buffer. After pressing the Enter key, the program can use the characters entered by the user, so scanf() and getchar() are also buffered from the input stream. The values ​​in the area, and people often have the illusion that they are taking values ​​from the keyboard buffer. In fact, the data is taken directly from the input stream buffer by the cin function, so when there is residual data in the buffer, the cin function will directly read the residual data without requesting keyboard input.
The 10 here is exactly the newline character '' entered by the Enter key. When the read data encounters the newline character '', the newline character will be read into the input stream buffer together, so the first time the input is accepted, the character is removed. Will leave the character '', so the second time will be taken directly from the buffer.
Why do you have a buffer? First, saving a number of characters as one block saves time by sending these characters one by one. Second, if the user hits the wrong character, the error can be corrected directly through the keyboard. When the Enter key is finally pressed, the correct input is transmitted. Although the benefits of the input buffer are numerous, there is no buffer input required in some interactive programs. For example, in a game, if you want to execute a corresponding command by pressing a key, buffered input and unbuffered input each have their own use, but this book assumes that all inputs are buffered inputs.
Buffers fall into two categories: fully buffered I/O and row buffered I/O. Fully buffered input refers to flushing the buffer when the buffer is filled. The content is sent to the destination, usually in the file input. The size of the buffer depends on the system, and the common size is 512 bytes and 4096 bytes. Line buffer I/O refers to flushing the buffer when a newline occurs. The keyboard input is usually the line buffer input, so the buffer is flushed after the Enter key is pressed.
Getchar() and scanf()Getchar() reads each character, including spaces, tabs, and newlines; scanf() skips spaces, tabs, and newlines when reading numbers. Although both of these functions work well, they cannot be mixed.
Although the parameter ch of putchar() is defined as an int type, it essentially receives a char type character, because inside putchar(), the system will cast ch to char type before using it. If the character output is successful, putchar() returns the output character ((char)ch) instead of the input parameter ch; if unsuccessful, it returns the predefined constant EOF (end of file), EOF is a Integer.
Getchar() has no input parameters, and its return value is int, not char. Here you need to distinguish between valid data in the file and the input terminator. When there is a character readable, getchar() does not return the end-of-file EOF, so
Ch = getchar() != EOF // Â Equivalent to ch = (getchar() != EOF)
The value is true and the variable ch is assigned the value 1.
When the program has no input, getchar() returns the file end character EOF, that is, the expression evaluates to false. At this time, the variable ch is assigned a value of 0, and the program ends. If the return value of the getchar() function is defined as an int, both the possible characters can be stored, and the end-of-file EOF can be stored. The example of copying the input to the output is shown in Listing 1.36.
Listing 1.36: Copying input to the output sample program
1 #include
2 int main(int argc, char *argv[])
3 {
4 int ch;
5
6 while((ch = getchar()) != EOF){
7 putchar(ch);
8 }
9 return 0;
10 }
Of course, you can also use the alternative idiom of getchar() to replace Listing 1.36(6):
While((ch = getchar()) != '')
A character to be read is compared with a newline character. If the test result is true, the loop body is executed, then the test loop condition is repeated, and then a new character is read, and getchar() is used to search for characters and skip character equivalents. . such as:
While((ch = getchar()) == ' ')
When the loop terminates, the variable ch will contain the first non-null character encountered by getchar().
Do-whileThe do-while loop is much smaller than the for and while loops because it requires at least one loop body to be executed, and at the end of the code instead of starting the conditional loop test. Logical conditions should appear before the code they "protect", which is how if, while, and for work. Usually the habit of reading code is from front to back. When using a do/while loop, you need to read this code twice. At the same time, this method is incorrect in many cases, such as:
Do{
? ch = getchar();
? putchar(ch);
? }while(ch != EOF);
Since the test was placed after the call to putchar(), the code writes one more character unreasonably. It is only correct to use a do-while loop if a loop body must be executed at least once.
Another confusing thing is the contiune statement in the do/while loop:
Do{
Continue;
}while(false);
Will it cycle forever or only once? Although it will only cycle once, most people will think about it. Bjarne Stroustrup, the pioneer of C++, said, "The do statement is the source of errors and confusion. I tend to put the conditions in front of what I can see and avoid using the do statement."
With the improvement of people's quality of life, people's lifestyles have also become varied. Different kinds of recreational products are starting to appear in people's lives, such as electronic cigarettes. The emergence of e-cigarettes represents a part of young people's thinking and means that electronic products are beginning to show a trend towards diversity.
simply replace the pods. The Pod system uses an integrated pod rather than a tank for higher nicotine strength and provides low power traction. the Pod system is rechargeable and has a longer life and higher battery capacity than disposable electronic cigarettes.
Our company Pod system has a built-in 380mAh battery and a USB charging port on the bottom. In comparison, the Pod system has a built-in battery of only 180mAh, but the Pod system charges much faster.
Our electronic cigarettes are of rechargeable construction. The first time you use the charger to charge, it is recommended to use up the remaining power before filling up, this is to ensure the performance of the battery.
Vape Pod System Oem,Vape Pod Oem,Close Pod Oem,Thc Pod Disposable
Shenzhen MASON VAP Technology Co., Ltd. , https://www.cbdvapefactory.com